Replacing the drawing title block in Autodesk Inventor with a single click. Using Inventors API its possible to replace the block on an old drawing in a fraction of a second, simply by copying the block from the current template and pasting it onto the old drawing.
Why replace the title block?
When everything is fresh and new, the need to replace the title block might seem unnecessary. However, as time progresses, details like the company address, logo, or other information stored in the title block might change. At this point, you might find yourself faced with potentially hundreds of drawings that require the title block to be updated.
The manual process of updating the title block involves opening both the old drawing and a new drawing with the updated title block, copying and pasting the block from the new one to the old one, and then deleting the old block before inserting the new one on each sheet.
However, this manual process is slow and tedious. How can we expedite it? By utilizing Inventor’s API, whether through a macro, iLogic rule, or .NET program, it becomes possible to execute all necessary actions with a single click, completing the task in less than a second.
The program
This program utilizes a donor drawing (currently set to C:/temp/New Template.idw
) and copies the drawing title block to the presently active drawing. Subsequently, it deletes the existing title block and inserts the new one on each sheet. To utilize this code, you’ll need to set the path to your desired donor drawing, likely your current template. The code copies the first listed title block; if your drawing contains more than one, you may need to adjust the code accordingly.
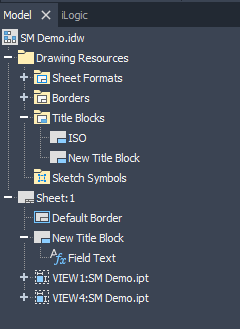
Sub New_Title_Block()
Dim oDoc As DrawingDocument
Set oDoc = ThisApplication.ActiveDocument
'disable deferred updates
If oDoc.DrawingSettings.DeferUpdates = True Then oDoc.DrawingSettings.DeferUpdates = False
oDoc.Update2 (True)
'get doner drawing, open in background
Dim sDoc As DrawingDocument
Set sDoc = ThisApplication.Documents.Open("C:/temp/New Template.idw", False)
'coppy data to new drawing
Dim sTitleBlock As TitleBlockDefinition
Set sTitleBlock = sDoc.TitleBlockDefinitions.Item(1)
Dim nTitleBlock As TitleBlockDefinition
Set nTitleBlock = sTitleBlock.CopyTo(oDoc)
'loop for every sheet in document
Dim oSheet As Sheet
For Each oSheet In oDoc.Sheets()
Call oSheet.Activate
'get rid of old title block
Call oSheet.TitleBlock.Delete
'add new title block
Call oSheet.AddTitleBlock(nTitleBlock)
Next
Call oDoc.Sheets.Item(1).Activate
Call sDoc.Close
End Sub
Dim oDoc As DrawingDocument = ThisApplication.ActiveDocument
'disable deferred updates
If oDoc.DrawingSettings.DeferUpdates = True Then oDoc.DrawingSettings.DeferUpdates = False
oDoc.Update2(True)
'get doner drawing, open in background
Dim sDoc As DrawingDocument = ThisApplication.Documents.Open("C:/temp/New Template.idw", False)
'coppy data to new drawing
Dim sTitleBlock As TitleBlockDefinition = sDoc.TitleBlockDefinitions.Item(1)
Dim nTitleBlock As TitleBlockDefinition = sTitleBlock.CopyTo(oDoc)
'loop for every sheet in document
Dim oSheet As Sheet
For Each oSheet In oDoc.Sheets()
oSheet.Activate()
'get rid of old title block
oSheet.TitleBlock.Delete()
'add new title block
Call oSheet.AddTitleBlock(nTitleBlock)
Next
oDoc.Sheets.Item(1).Activate()
sDoc.Close()
VB and C# both assume you have a global variable for the Inventor application called “_invApp
” if you need to set one up take a look at This page.
Sub New_Title_Block()
Dim oDoc As DrawingDocument = _invApp.ActiveDocument
'disable deferred updates
If oDoc.DrawingSettings.DeferUpdates = True Then oDoc.DrawingSettings.DeferUpdates = False
oDoc.Update2(True)
'get doner drawing, open in background
Dim sDoc As DrawingDocument = _invApp.Documents.Open("C:/temp/New Template.idw", False)
'coppy data to new drawing
Dim sTitleBlock As TitleBlockDefinition = sDoc.TitleBlockDefinitions.Item(1)
Dim nTitleBlock As TitleBlockDefinition = sTitleBlock.CopyTo(oDoc)
'loop for every sheet in document
Dim oSheet As Sheet
For Each oSheet In oDoc.Sheets()
oSheet.Activate()
'get rid of old title block
oSheet.TitleBlock.Delete()
'add new title block
Call oSheet.AddTitleBlock(nTitleBlock)
Next
oDoc.Sheets.Item(1).Activate()
sDoc.Close()
End Sub
public static void New_Title_Block()
{
DrawingDocument oDoc = (DrawingDocument)_invApp.ActiveDocument;
//disable deferred updates
if (oDoc.DrawingSettings.DeferUpdates) { oDoc.DrawingSettings.DeferUpdates = false; };
oDoc.Update2(true);
//get doner drawing, open in background
DrawingDocument sDoc = (DrawingDocument)_invApp.Documents.Open("C:/temp/New Template.idw", false);
//coppy data to new drawing
TitleBlockDefinition sTitleBlock = sDoc.TitleBlockDefinitions[1];
TitleBlockDefinition nTitleBlock = sTitleBlock.CopyTo((_DrawingDocument)oDoc);
//loop for every sheet in document
foreach (Sheet oSheet in oDoc.Sheets)
{
oSheet.Activate();
//get rid of old title block
oSheet.TitleBlock.Delete();
//add new title block
oSheet.AddTitleBlock(nTitleBlock);
}
oDoc.Sheets[1].Activate();
sDoc.Close();
}
What’s happening?
oDoc
oDoc
is the variable used to keep track of the drawing we whish to update. It is set to a ‘DrawingDocument
‘, and the currently open document in Inventor is assigned to it.
deferred updates
This setting enables Inventor to open large drawings more swiftly by avoiding update checks. However, when we intend to make alterations to the drawing, this setting should be disabled. An ‘if’ statement is employed to verify if deferred updates are active. If it is, the code sets the value to ‘False,’ turning it off. Additionally, we instruct Inventor to update the drawing in case any modifications need to be applied.
sDoc
Next, the donor drawing is opened, but only in the background. To make this function, you’ll need to adjust the string to the location of your current template. Opening files in the background accelerates the process as it relieves Inventor from processing the graphics. It’s important to remember to close the drawing when finished, as Inventor will not automatically close the document on it’s own.
Copying the title block
In the donor drawing, we select the first title block. If there are multiple title blocks, you might want to modify this value to select the most recent version. Then, the title block is copied to the new drawing. We need to keep track of it by storing it in the variable ‘nTitleBlock
‘ as this action has only added it to the drawing resources
loop
Next, the new title block needs to be added to each sheet. To accomplish this, we utilize a ‘for each’ statement to iterate through every sheet in the drawing. This approach ensures consistency whether you have 1 or 100 sheets. Within each sheet, the process involves replacing the drawing title block by first deleting the existing one and then adding the new one stored in ‘nTitleBlock’.
Tidy up
Once complete we set the currently active sheet back to the first for the convenience of the user. Then, it’s time to close the donor drawing.
Background documents
When opening files in the background, the responsibility falls on your program to close them again. If your program crashes before reaching the ‘close’ command, the file will remain open in Inventor. However, in this scenario, as no changes are made to the donor drawing, it has no significant impact.
To verify hidden files, inspect the bottom right corner. When all active documents are closed, this number should consistently be zero. If it’s not, you can manually close background files by clicking on the hamburger menu above and selecting ‘Close All’.
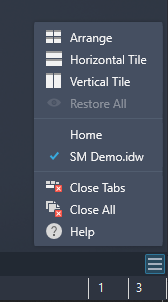
Leave a Reply