Exporting a flat pattern DXF and other file types with Inventor macro.
This project will be export flat pattern DXF of a sheet metal part with a single click from the drawing. The project can easily be modified if you whish to add support for other file types such as a STP file or PDF of the drawing.
Macro basics
Inventor macros utilize Microsoft VBA (Visual Basic for Applications), offering the capability to connect with Inventor’s API as well as other VBA-supporting applications. You can access the VBA Editor in the Tools tab of Inventor.
Within the Project section, you will discover the application project and projects for any presently open documents. The projects dictate where the files are saved, to Inventor or the document. For the scope of this project, we aim to utilize the application project.
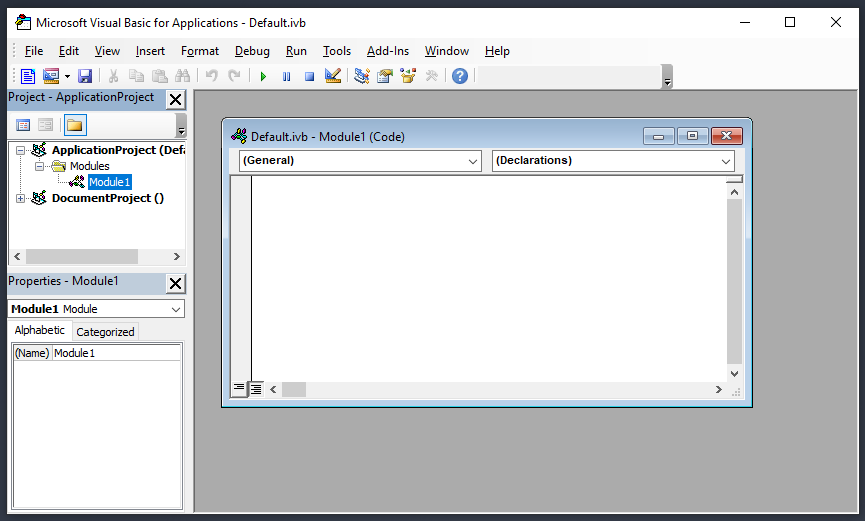
The Code
This macro is designed to retrieve the currently open drawing document, acquire the user-specified folder for saving the file , and gather the name of the drawing. It proceeds to search through all the referenced files, sorting them by file type. If it identifies a sheet metal file among the references, it attempts to export the flat pattern DXF from the background. In case of failure, it initiates a secondary attempt by opening the file and trying again. If no sheet metal file is found, it generates a message box to inform the user.
Sub Export()
'get the drawing
Dim dDoc As DrawingDocument
Set dDoc = ThisApplication.ActiveDocument
'set the output directory
Dim Folder As String
Folder = "C:/temp"
'get the drawing file name
Dim FileName As String
'check if aDoc has been saved
If dDoc.FileSaveCounter = 0 Then
FileName = dDoc.DisplayName
Else
'Get the file name without directory or file extention
Dim File As String
File = dDoc.FullFileName
FileName = Strings.Right(File, (Len(File) - InStrRev(File, "\")))
FileName = Strings.Left(FileName, InStrRev(FileName, ".") - 1)
End If
Dim pDoc As PartDocument
Dim smDoc As PartDocument
Dim aDoc As AssemblyDocument
'sort the referenced documents by type
For Each rDoc In dDoc.ReferencedDocuments
Select Case rDoc.SubType
Case "{4D29B490-49B2-11D0-93C3-7E0706000000}" 'part
Set pDoc = rDoc
Case "{9C464203-9BAE-11D3-8BAD-0060B0CE6BB4}" 'sheet metal
Set smDoc = rDoc
Case "{E60F81E1-49B3-11D0-93C3-7E0706000000}" 'assembly document sub-types
Set aDoc = rDoc
End Select
Next
'DXF file version
Dim sOut As String
sOut = "FLAT PATTERN DXF?AcadVersion=2004"
'Export Flat pattern
If Not smDoc Is Nothing Then
' set the DataIO object.
Dim oDataIO
Set oDataIO = smDoc.ComponentDefinition.DataIO
On Error GoTo Oops
Call oDataIO.WriteDataToFile(sOut, Folder + "\" + FileName + ".dxf")
Continue:
dxf_sucsess = True
End If
'inform user of error
If Not dxf_sucsess Then
MsgBox "Dxf Failed"
End If
Exit Sub
'if export failed try again with file open
Oops:
Set smDoc = ThisApplication.Documents.Open(smDoc.FullFileName, False)
Call oDataIO.WriteDataToFile(sOut, Folder + "\" + FileName + ".dxf")
Call smDoc.Close
GoTo Continue:
End Sub
Backdown
- Lines 3-4, the variable ‘dDoc’ is employed to retain a reference to the presently active drawing, enabling quick retrieval without repeatedly querying Inventor for the active drawing.
- Lines 7-8, the output directory is assigned; it’s essential for this folder to already exist. You may set this to anywhere you prefer.
- Lines 11-21 handle the acquisition of the drawing’s file name. As the display name doesn’t necessarily match the file name, it’s optimal to use the file name when the drawing is saved and the display name when it’s not. When using the file name, it’s necessary to remove the directories and file extension to prevent issues during saving.
- Lines 24-38 sort the referenced documents by three file types. Presently, the program doesn’t utilize part or assembly files, and this code serves as an illustrative example. Sorting is achieved by checking the GUID of the file subtype and comparing it to the three expected file types. If other types are encountered, they are simply ignored. If you whish to use other sub types check out this page.
- Lines 41-42 establish the DXF options, which, in their current state, match the default options for Inventor.
- Lines 45-53 export flat pattern DXF, first verifying the presence of a sheet metal part. Subsequently, a DataIO object is set up for the part, and an attempt is made to export. If the flat pattern is not currently up to date on the part, this process may fail, as the flat pattern cannot update in the background. In such cases, the program proceeds to line 62.
- Lines 56-58, in case no sheet metal part is found, trigger a message box to inform the user.
- Lines 62-66, if the flat pattern export fails, the program retries the export, this time with the part open. If successful, it then returns to line 51.
Some assumptions are made
The program assumes that the currently active file is a drawing, and if not, it will instantly crash. An enhancement could involve adding a check similar to how it sorts the referenced documents. This would empower the programmer to incorporate a message box to inform the user or modify the functionality based on different file types.
It’s important to note that the program can only handle one of each file type. If a drawing has two referenced sheet metal parts, only one will get exported. While handling multiple copies could be achieved using arrays, this feature hasn’t been implemented to maintain the simplicity of the program, given that the scenario requiring such functionality has not arisen.
Adding other file types
Incorporating 3D file types, like STP, is a straightforward process. Just insert the following code below line 39. This code exports only one file but follows a priority system. For instance, if the drawing contains both an assembly and a sheet metal part, the program prioritizes the assembly, assuming the sheet metal part is present within the assembly. Adapting it for other file types is as simple as replacing ‘.stp
‘ with any other supported file type.
If aDoc IsNot Nothing Then
Call aDoc.SaveAs(Folder + "\" + FileName + ".stp", True)
stp_Sucsess = True
ElseIf pDoc IsNot Nothing Then
Call pDoc.SaveAs(Folder + "\" + FileName + ".stp", True)
stp_Sucsess = True
ElseIf smDoc IsNot Nothing Then
Call smDoc.SaveAs(Folder + "\" + FileName + ".stp", True)
stp_Sucsess = True
End If
Generating a PDF of the drawing is a slightly more complex task. The PDF generator is an add-on for Inventor, and to utilize it, we must fetch the add-on by its GUID. Once we have a reference to it, we just need to specify the desired formatting for the PDF. This section can be added after line 22.
Dim oPDFTrans As TranslatorAddIn
Set oPDFTrans = ThisApplication.ApplicationAddIns.ItemById("{0AC6FD96-2F4D-42CE-8BE0-8AEA580399E4}")
Dim oContext As TranslationContext
Set oContext = ThisApplication.TransientObjects.CreateTranslationContext
Dim oOptions As NameValueMap
Set oOptions = ThisApplication.TransientObjects.CreateNameValueMap
oContext.Type = IOMechanismEnum.kFileBrowseIOMechanism
oOptions.Value("Sheet_Range") = PrintRangeEnum.kPrintAllSheets
oOptions.Value("Remove_Line_Weights") = 0.25
Dim oData As DataMedium
Set oData = ThisApplication.TransientObjects.CreateDataMedium
oData.FileName = Folder + "\" + FileName + ".pdf"
'Create the PDF file.
Call oPDFTrans.SaveCopyAs(dDoc, oContext, oOptions, oData)
Add macro to your Ribbon
If you don’t want to go into the editor every time you wish to run the macro, you can always add it to the ribbon at the top of the screen.
To add a macro to the ribbon, simply right-click and select ‘Customize.’ In the left-hand column, change the drop-down box to ‘Macros,’ select the macro you want, and add it to the Ribbon.
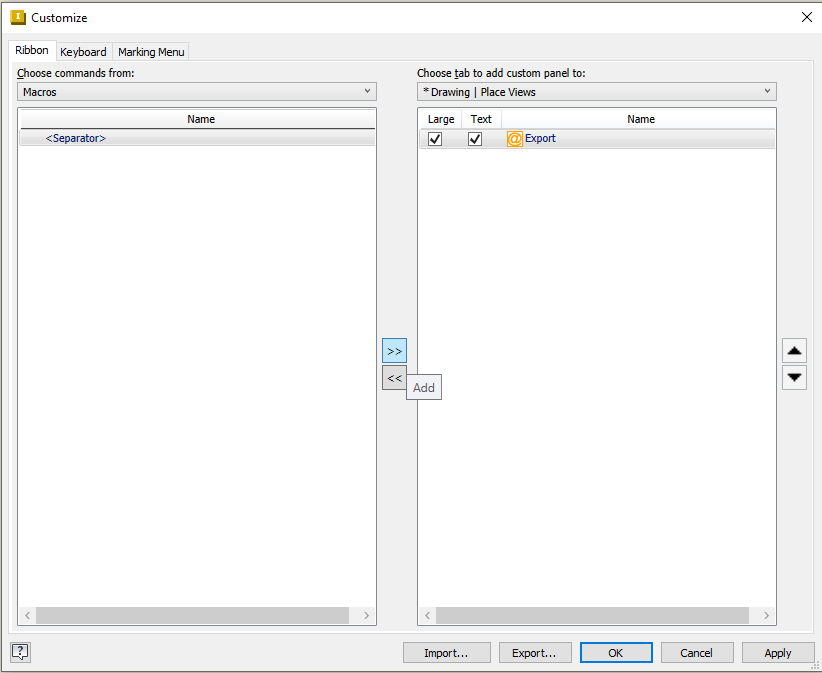
Leave a Reply